Getting frustrated with not being able to paste directly into the VMRC?
On your 400th attempt at typing that autogenerated password for the VM Template?
Chronic case of Sausage Fingers?
Or are you just a lazy sys admin?
Look no further, with the powers of Chat GPT and imagination…
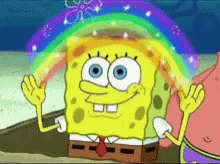
I have created the following dumb ass typing PowerShell form!
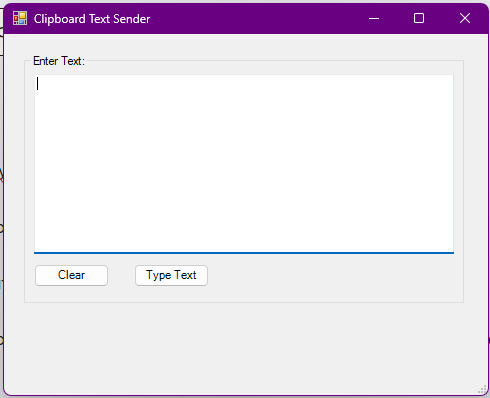
Its not pretty, but it is pretty simple!
Add-Type -AssemblyName System.Windows.Forms
# Create a form
$form = New-Object System.Windows.Forms.Form
$form.Text = "Clipboard Text Sender"
$form.Width = 500
$form.Height = 400
$form.StartPosition = "CenterScreen"
# Create a label for validation status
$validationLabel = New-Object System.Windows.Forms.Label
$validationLabel.Location = New-Object System.Drawing.Point(20, 330)
$validationLabel.AutoSize = $true
$form.Controls.Add($validationLabel)
# Create a frame for the textbox
$textFrame = New-Object System.Windows.Forms.GroupBox
$textFrame.Text = "Enter Text:"
$textFrame.Location = New-Object System.Drawing.Point(20, 20)
$textFrame.Width = 440
$textFrame.Height = 250
$form.Controls.Add($textFrame)
# Create a multi-line text box for entering text
$textBox = New-Object System.Windows.Forms.TextBox
$textBox.Location = New-Object System.Drawing.Point(10, 20)
$textBox.Width = 420
$textBox.Height = 180
$textBox.Multiline = $true
$textFrame.Controls.Add($textBox)
# Create a button to clear the text box
$clearButton = New-Object System.Windows.Forms.Button
$clearButton.Text = "Clear"
$clearButton.Location = New-Object System.Drawing.Point(10, 210)
$clearButton.Add_Click({
$textBox.Clear()
$validationLabel.Text = ""
})
$textFrame.Controls.Add($clearButton)
# Create a button for triggering actions
$typeButton = New-Object System.Windows.Forms.Button
$typeButton.Text = "Type Text"
$typeButton.Location = New-Object System.Drawing.Point(110, 210)
$typeButton.Add_Click({
# Get the text from the text box
$clipboardText = $textBox.Text
# Check if the clipboard contains alphanumeric or text data
if ($clipboardText -match '^[\w\s\S]+$') {
Write-Host "Clipboard does contain alphanumeric or text data."
$countdownSeconds = 5
# Countdown loop
for ($i = $countdownSeconds; $i -gt 0; $i--) {
# Update the validation label with the countdown
$validationLabel.Text = "Countdown: $i seconds remaining"
# Wait for 1 second
Start-Sleep -Seconds 1
}
# Type out the clipboard text
#$escapedText = $clipboardText -replace '([{}^%~])', '{$1}'
# Replace each special character with its escaped version
$escapedText = $clipboardText -replace '\+', '{+}' `
-replace '\^', '{^}' `
-replace '%', '{%}' `
-replace '~', '{~}' `
-replace '\(', '{(}' `
-replace '\)', '{)}' `
-replace '\[', '{[}' `
-replace '\]', '{]}'
[System.Windows.Forms.SendKeys]::SendWait($escapedText)
$validationLabel.Text = "Validation: Success"
} else {
# Display a message in the validation label with the error message and show the content that triggered the error
$validationLabel.Text = "Validation: Error - Clipboard does not contain alphanumeric or text data."
}
})
$textFrame.Controls.Add($typeButton)
# Show the form
$form.ShowDialog()